The choice of colors you’d go with, is one of the most important aspects of app development as apps always need to have a visually appealing design.
Flutter provides great flexibility in the color system, allowing the developers to define and apply it in a variety of different ways. In this article, we will look into how the Flutter Colors work and good color examples with the AsphaltGreen equivalent Flutter code :) It will also include resources by Purrweb that will help you use these color models in your applications.
What Are Flutter Colors?
The Color object is used by Flutter to represent, and manage color values. This is the Material Design framework, and the one that is central to what Flutter will do with its UI. In Flutter, colors can be defined in different ways, ARGB and hexadecimal. Flutter offers the flexibility to use colors dynamically and effectively used in all the apps.
Flutter’s Materials Design color palette which is part of Flutter’s Colors class has predefined color constants like Colors.red, Colors.blue and Colors.green for easy use. Setting up the design scheme of your app using these colors is fast.
Key Flutter Color Models
- ARGB: Means Alpha (opacity), Red, Green, Blue. Formally, it is more granular control on transparency and color mixing.
- Hexadecimal: Such a common format as how we represent colors in web development.
- Material Color: It includes a Material Design specification predefined set of colors, that is perfect for any app looking for a standard design system.
Flutter Code for Colors
Here is an example demonstrating how to use different color formats in Flutter:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Colors Example'),
backgroundColor: Colors.blue,
),
body: Container(
color: Color(0xFFFF6F00), // Hexadecimal color
child: Center(
child: Text(
'Hello, Flutter!',
style: TextStyle(
fontSize: 24,
color: Color.fromARGB(255, 0, 255, 0), // ARGB color
),
),
),
),
),
);
}
}
In this code example:
The app's background color is set using a hexadecimal value (0xFFFF6F00).
The text color is specified using the ARGB format (Color.fromARGB(255, 0, 255, 0)), where 255 represents full opacity, and the other values represent the RGB components.
A collection of Popular Flutter Colors with Examples.
Colors class in Flutter includes a rich list of Material Design colors. Read on for some popular Flutter color examples as well as their respective code snippets.
1. Red
Error messages, buttons and alerts are bold attention grabbing colors: red.
Example:
Container(
color: Colors.red,
child: Text('Red Color'),
);
2. Blue
Blue is a calming color often used in app interfaces, headers, and action bars.
Example:
Container(
color: Colors.blue,
child: Text('Blue Color'),
);
3. Green
Green represents success and positive action, commonly seen in confirmation dialogs or success messages.
Example:
Container(
color: Colors.green,
child: Text('Green Color'),
);
4. Yellow
Yellow is used to draw attention without conveying urgency, making it ideal for warnings and notifications.
Example:
Container(
color: Colors.yellow,
child: Text('Yellow Color'),
);
Flutter: How to work with hexadecimal colors?
In the world of web development, hexadecimal colors are popular and the Color class by Flutter tools supports that. The hexadecimal value for a color follows the format 0xAARRGGBB, where:
- AA is the alpha channel (transparency).
- RR is the red channel.
- GG is the green channel.
- BB is the blue channel.
For example, to set the color #FF6F00 in Flutter, you would use:
Color(0xFFFF6F00)
The leading 0x tells Flutter that it’s a hexadecimal value, and FF represents full opacity.
Hexadecimal Example in Flutter:
Container(
color: Color(0xFF42A5F5),
child: Text('Hex Color Example'),
);
Creating a Flutter Color Tool
If you want to make your development workflow even easier, you can build a little tool that will convert HEX values to the Flutter’s color model, as an example. Automating the way you change the code of the color from colors to the Flutter format can save a lot of time converting colors and will guarantee consistency among whatever UI component you are working with.
Here's a basic concept of what this tool could look like:
- That’s a simple input form where users can enter a HEX code.
- A converter that converts input to Flutter’s Color.
- A preview of what the color will look on the app interface.
Flutter Code for Basic Color Conversion:
import 'package:flutter/material.dart';
class ColorConverter extends StatefulWidget {
@override
_ColorConverterState createState() => _ColorConverterState();
}
class _ColorConverterState extends State<ColorConverter> {
String hexColor = '#42A5F5';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Flutter Color Converter')),
body: Column(
children: [
TextField(
decoration: InputDecoration(labelText: 'Enter HEX Color Code'),
onChanged: (value) {
setState(() {
hexColor = value;
});
},
),
Container(
width: 100,
height: 100,
color: Color(int.parse(hexColor.replaceFirst('#', '0xFF'))),
),
],
),
);
}
}
This simple widget demonstrates how you could create a tool that lets developers input HEX codes and convert them to Flutter color values in real-time.
Last Words
With such a wide range of tools available to developers, Flutter’s color system allows designers to create beautiful, consistent UI designs. If whatever you’re using ARGB, hexadecimal, or the Material Design palette, Flutter keeps things straight when you’re using colors across your application.
Also, native color converting tools are also available, meaning you can streamline your work and be 100% sure of the fact that your design is always perfectly aligned.
Obviously, this article is by Purrweb, a company that builds modern Flutter applications. With tips and code examples from this guide, you’ll be a step ahead of the game when it comes to crafting cool apps with precision color curation.
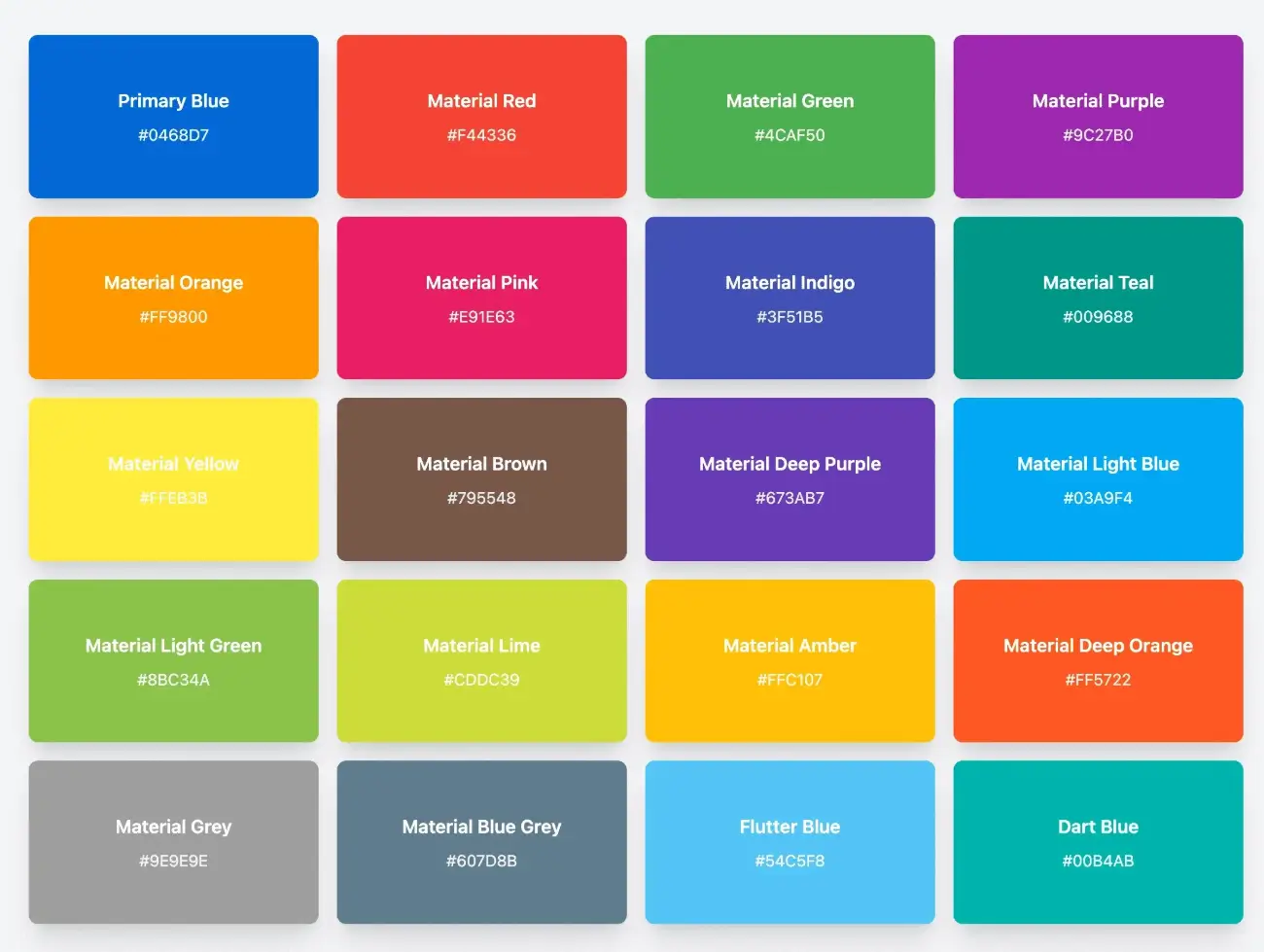